javascript to disable browser back button
In this example i'm explaining how to disable browser's back button to avoid user going to previous page by clicking on back button of browser, for this we need to use javascript to prevent user navigating to previous page by hitting back button.
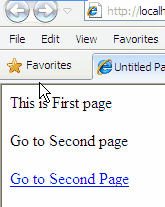
Just put this javascript on the html section of aspx page above head section
We need to put it on the html section of the page which we want to prevent user to visit by hitting the back button
Complete code of the page looks like this
If you want to disable back button using code behind of aspx page,than you need to write below mentioned code
C# code behind
We can also achieve this by disabling browser caching or cache by writing this line of code either in Page_load event or in Page_Init event
Download the sample code attached

In this example i'm explaining how to disable browser's back button to avoid user going to previous page by clicking on back button of browser, for this we need to use javascript to prevent user navigating to previous page by hitting back button.
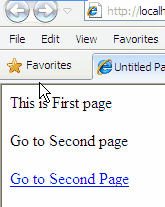
Just put this javascript on the html section of aspx page above head section
<script type = "text/javascript" >
function disableBackButton()
{
window.history.forward();
}
setTimeout("disableBackButton()", 0);
</script>
function disableBackButton()
{
window.history.forward();
}
setTimeout("disableBackButton()", 0);
</script>
We need to put it on the html section of the page which we want to prevent user to visit by hitting the back button
Complete code of the page looks like this
<%@ Page Language="C#" AutoEventWireup="true"
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
<script type = "text/javascript" >
function disableBackButton()
{
window.history.forward();
}
setTimeout("disableBackButton()", 0);
</script>
</head>
<body onload="disableBackButton()">
<form id="form1" runat="server">
<div>
This is First page <br />
<br />
Go to Second page
<br />
<br />
<asp:LinkButton ID="LinkButton1" runat="server"
PostBackUrl="~/Default2.aspx">Go to Second Page
</asp:LinkButton></div>
</form>
</body>
</html>
CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head runat="server">
<title>Untitled Page</title>
<script type = "text/javascript" >
function disableBackButton()
{
window.history.forward();
}
setTimeout("disableBackButton()", 0);
</script>
</head>
<body onload="disableBackButton()">
<form id="form1" runat="server">
<div>
This is First page <br />
<br />
Go to Second page
<br />
<br />
<asp:LinkButton ID="LinkButton1" runat="server"
PostBackUrl="~/Default2.aspx">Go to Second Page
</asp:LinkButton></div>
</form>
</body>
</html>
If you are using firefox then use <body onunload="disableBackButton()"> instead of onload
If you want to disable back button using code behind of aspx page,than you need to write below mentioned code
C# code behind
1
protected
override
void
OnPreRender(EventArgs e)
2
{
3
base
.OnPreRender(e);
4
string
strDisAbleBackButton;
5
strDisAbleBackButton =
"<script language="
javascript
">\n"
;
6
strDisAbleBackButton +=
"window.history.forward(1);\n"
;
7
strDisAbleBackButton +=
"\n</script>"
;
8
ClientScript.RegisterClientScriptBlock(
this
.Page.GetType(),
"clientScript"
, strDisAbleBackButton);
9
}
We can also achieve this by disabling browser caching or cache by writing this line of code either in Page_load event or in Page_Init event
protected void Page_Init(object Sender, EventArgs e)
{
Response.Cache.SetCacheability(HttpCacheability.NoCache);
Response.Cache.SetExpires(DateTime.Now.AddSeconds(-1));
Response.Cache.SetNoStore();
}
Doing this,user will get the page has expired message when hitting back button of browser{
Response.Cache.SetCacheability(HttpCacheability.NoCache);
Response.Cache.SetExpires(DateTime.Now.AddSeconds(-1));
Response.Cache.SetNoStore();
}
Download the sample code attached

No comments:
Post a Comment