Tuesday 30 October 2012
Monday 29 October 2012
Common Table Expressions
Syntax:
-----GENERAL METHOD
SELECT a.Payment_ID,a.Purchase_Order_ID,Payment_Date,Actual_Amount,a.Payment_Amount,
Bank_Name,[Cheque_No/DD_No/Card_No],
Created_On,Created_By,Modified_On,Modified_By,Is_Active,Is_Deleted
FROM
(SELECT MAX(Payment_ID) Payment_ID,Purchase_Order_ID,
--ROW_NUMBER() OVER(PARTITION BY Purchase_Order_ID ORDER BY Purchase_Order_ID) Purchase_Order_ID ,
SUM(Payment_Amount) Payment_Amount
FROM Uams.AMS_Payment
GROUP BY purchase_Order_ID) a
JOIN
Uams.AMS_Payment P
ON a.Payment_ID=p.Payment_ID
-----WITH COMMON TABLE EXPRESSION
WITH Cte_Payment(Payment_ID,Purchase_Order_ID,Payment_Amount)
AS
(SELECT MAX(Payment_ID) Payment_ID,Purchase_Order_ID,
--ROW_NUMBER() OVER(PARTITION BY Purchase_Order_ID ORDER BY Purchase_Order_ID) Purchase_Order_ID ,
SUM(Payment_Amount) Payment_Amount
FROM Uams.AMS_Payment
GROUP BY purchase_Order_ID)
SELECT Cte_Payment.Payment_ID,Cte_Payment.Purchase_Order_ID,Payment_Date,Actual_Amount,Cte_Payment.Payment_Amount,
Bank_Name,[Cheque_No/DD_No/Card_No],
Created_On,Created_By,Modified_On,Modified_By,Is_Active,Is_Deleted
FROM Cte_Payment
JOIN
Uams.AMS_Payment P
ON Cte_Payment.Payment_ID=p.Payment_ID
[WITH <common_table_expression> [,...]]
<common_table_expression>::=
cte_name [(column_name [,...])]
AS (cte_query)
CTE (Common Table Expression):
The CTE is one of the essential features in the sql server 2005.It just store the result as temp result set. It can be access like normal table or view. This is only up to that scope.
The syntax of the CTE is the following.
WITH name (Alias name of the retrieve result set fields)
AS
(
//Write the sql query here
)
SELECT * FROM name
Here the select statement must be very next to the CTE. The name is mandatory and the argument is an optional. This can be used to give the alias to the retrieve field of the CTE.
CTE 1: Simple CTE
WITH ProductCTE
AS( SELECT ProductID AS [ID],ProductName AS [Name],CategoryID AS [CID],UnitPrice AS [Price]
FROM Products
)SELECT * FROM ProductCTE
Here all the product details like ID, name, category ID and Unit Price will be retrieved and stored as temporary result set in the ProductCTE.
This result set can be retrieved like table or view.
CTE2:Simple CTE with alias
WITH ProductCTE(ID,Name,Category,Price)AS( SELECT ProductID,ProductName,CategoryID,UnitPrice
FROM Products
)SELECT * FROM ProductCTE
Here there are four fieds retrieves from the Products and the alias name have given in the arqument to the CTE result set name.
It also accepts like the following as it is in the normal select query.
WITH ProductCTE
AS( SELECT ProductID AS [ID],ProductName AS [Name],CategoryID AS [CID],UnitPrice AS [Price]
FROM Products
)SELECT * FROM ProductCTE
CTE 3: CTE joins with normal table
The result set of the CTE can be joined with any table and also can enforce the relationship with the CTE and other tables.
WITH OrderCustomer
AS( SELECT DISTINCT CustomerID FROM Orders
)SELECT C.CustomerID,C.CompanyName,C.ContactName,C.Address+', '+C.City AS [Address] FROM Customers C INNER JOIN OrderCustomer OC ON OC.CustomerID = C.CustomerID
Here the Ordered Customers will be placed in the CTE result set and it will be joined with the Customers details.
CTE 4: Multiple resultsets in the CTE
WITH MyCTE1
AS( SELECT ProductID,SupplierID,CategoryID,UnitPrice,ProductName FROM Products
),
The CTE is one of the essential features in the sql server 2005.It just store the result as temp result set. It can be access like normal table or view. This is only up to that scope.
The syntax of the CTE is the following.
WITH name (Alias name of the retrieve result set fields)
AS
(
//Write the sql query here
)
SELECT * FROM name
Here the select statement must be very next to the CTE. The name is mandatory and the argument is an optional. This can be used to give the alias to the retrieve field of the CTE.
CTE 1: Simple CTE
WITH ProductCTE
AS( SELECT ProductID AS [ID],ProductName AS [Name],CategoryID AS [CID],UnitPrice AS [Price]
FROM Products
)SELECT * FROM ProductCTE
Here all the product details like ID, name, category ID and Unit Price will be retrieved and stored as temporary result set in the ProductCTE.
This result set can be retrieved like table or view.
CTE2:Simple CTE with alias
WITH ProductCTE(ID,Name,Category,Price)AS( SELECT ProductID,ProductName,CategoryID,UnitPrice
FROM Products
)SELECT * FROM ProductCTE
Here there are four fieds retrieves from the Products and the alias name have given in the arqument to the CTE result set name.
It also accepts like the following as it is in the normal select query.
WITH ProductCTE
AS( SELECT ProductID AS [ID],ProductName AS [Name],CategoryID AS [CID],UnitPrice AS [Price]
FROM Products
)SELECT * FROM ProductCTE
CTE 3: CTE joins with normal table
The result set of the CTE can be joined with any table and also can enforce the relationship with the CTE and other tables.
WITH OrderCustomer
AS( SELECT DISTINCT CustomerID FROM Orders
)SELECT C.CustomerID,C.CompanyName,C.ContactName,C.Address+', '+C.City AS [Address] FROM Customers C INNER JOIN OrderCustomer OC ON OC.CustomerID = C.CustomerID
Here the Ordered Customers will be placed in the CTE result set and it will be joined with the Customers details.
CTE 4: Multiple resultsets in the CTE
WITH MyCTE1
AS( SELECT ProductID,SupplierID,CategoryID,UnitPrice,ProductName FROM Products
),
MyCTE2
AS( SELECT DISTINCT ProductID FROM "Order Details"
)SELECT C1.ProductID,C1.ProductName,C1.SupplierID,C1.CategoryID FROM MyCTE1 C1 INNER JOIN MyCTE2 C2 ON C1.ProductID = C2.ProductID
Here, there are two result sets that will be filtered based on the join condition.
CTE 5: Union statements in the CTE
WITH PartProdCateSale
AS(SELECT ProductID FROM Products WHERE CategoryID = (SELECT CategoryID FROM Categories WHERE CategoryName='Condiments')UNION ALL
SELECT ProductID FROM Products WHERE CategoryID = (SELECT CategoryID FROM Categories WHERE CategoryName='Seafood')
)SELECT OD.ProductID,SUM(OD.UnitPrice*OD.Quantity) AS [Total Sale] FROM "Order Details" OD INNER JOIN PartProdCateSale PPCS ON PPCS.ProductID = OD.ProductID
GROUP BY OD.ProductID
Normally when we combine the many result sets we create table and then insert into that table. But see here, we have combined with the union all and instead of table, here CTE has used.
CTE 6: CTE with identity column
WITH MyCustomCTE
AS ( SELECT CustomerID,row_number() OVER (ORDER BY CustomerID) AS iNo FROM
Customers
)SELECT * FROM MyCustomCTE
AS( SELECT DISTINCT ProductID FROM "Order Details"
)SELECT C1.ProductID,C1.ProductName,C1.SupplierID,C1.CategoryID FROM MyCTE1 C1 INNER JOIN MyCTE2 C2 ON C1.ProductID = C2.ProductID
Here, there are two result sets that will be filtered based on the join condition.
CTE 5: Union statements in the CTE
WITH PartProdCateSale
AS(SELECT ProductID FROM Products WHERE CategoryID = (SELECT CategoryID FROM Categories WHERE CategoryName='Condiments')UNION ALL
SELECT ProductID FROM Products WHERE CategoryID = (SELECT CategoryID FROM Categories WHERE CategoryName='Seafood')
)SELECT OD.ProductID,SUM(OD.UnitPrice*OD.Quantity) AS [Total Sale] FROM "Order Details" OD INNER JOIN PartProdCateSale PPCS ON PPCS.ProductID = OD.ProductID
GROUP BY OD.ProductID
Normally when we combine the many result sets we create table and then insert into that table. But see here, we have combined with the union all and instead of table, here CTE has used.
CTE 6: CTE with identity column
WITH MyCustomCTE
AS ( SELECT CustomerID,row_number() OVER (ORDER BY CustomerID) AS iNo FROM
Customers
)SELECT * FROM MyCustomCTE
With T(Address, Name, Age) --Column names for Temporary table
AS
(
SELECT A.Address, E.Name, E.Age from Address A
INNER JOIN EMP E ON E.EID = A.EID
)
SELECT * FROM T --SELECT or USE CTE temporary Table
WHERE T.Age > 50
ORDER BY T.NAME
-----GENERAL METHOD
SELECT a.Payment_ID,a.Purchase_Order_ID,Payment_Date,Actual_Amount,a.Payment_Amount,
Bank_Name,[Cheque_No/DD_No/Card_No],
Created_On,Created_By,Modified_On,Modified_By,Is_Active,Is_Deleted
FROM
(SELECT MAX(Payment_ID) Payment_ID,Purchase_Order_ID,
--ROW_NUMBER() OVER(PARTITION BY Purchase_Order_ID ORDER BY Purchase_Order_ID) Purchase_Order_ID ,
SUM(Payment_Amount) Payment_Amount
FROM Uams.AMS_Payment
GROUP BY purchase_Order_ID) a
JOIN
Uams.AMS_Payment P
ON a.Payment_ID=p.Payment_ID
-----WITH COMMON TABLE EXPRESSION
WITH Cte_Payment(Payment_ID,Purchase_Order_ID,Payment_Amount)
AS
(SELECT MAX(Payment_ID) Payment_ID,Purchase_Order_ID,
--ROW_NUMBER() OVER(PARTITION BY Purchase_Order_ID ORDER BY Purchase_Order_ID) Purchase_Order_ID ,
SUM(Payment_Amount) Payment_Amount
FROM Uams.AMS_Payment
GROUP BY purchase_Order_ID)
SELECT Cte_Payment.Payment_ID,Cte_Payment.Purchase_Order_ID,Payment_Date,Actual_Amount,Cte_Payment.Payment_Amount,
Bank_Name,[Cheque_No/DD_No/Card_No],
Created_On,Created_By,Modified_On,Modified_By,Is_Active,Is_Deleted
FROM Cte_Payment
JOIN
Uams.AMS_Payment P
ON Cte_Payment.Payment_ID=p.Payment_ID
Sunday 28 October 2012
How to Create a Custom Control
1) Start a new Windows Forms Control Library project; see:
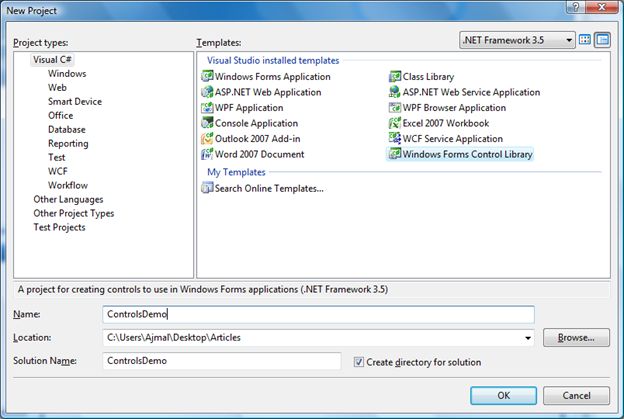
2) Remove UserControl1.cs which has been created by default and add a new class; see:
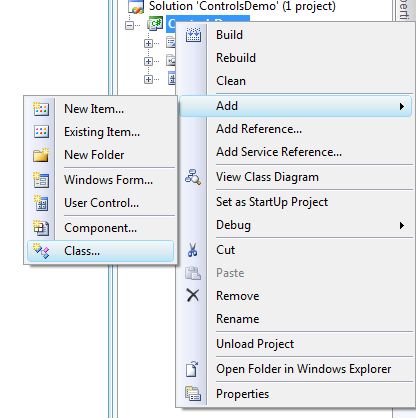
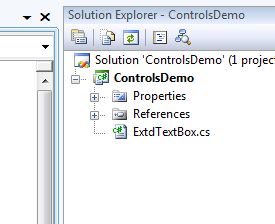
3)
Add the following namespaces to the new class (in our case its
ExtdTextBox.cs) and inherit the framework's TextBox control class:
using System.Windows.Forms;
using System.ComponentModel;
using System.Drawing;
class ExtdTextBox:TextBox
{
}
4) Let as add a feature for watermark text to our custom TextBox Control and let us give an option to the user to select the WaterMark Text color, WaterMark Text, WaterMark Font at design time which will be available as a Control property window of Visual Studio as shown below:
using System.Windows.Forms;
using System.ComponentModel;
using System.Drawing;
class ExtdTextBox:TextBox
{
}
4) Let as add a feature for watermark text to our custom TextBox Control and let us give an option to the user to select the WaterMark Text color, WaterMark Text, WaterMark Font at design time which will be available as a Control property window of Visual Studio as shown below:
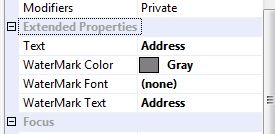
To
display properties in Visual Studio Tool bar GUI we need to add the
Browsable(true) attribute to the Properties to be displayed at design
time as shown below:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.ComponentModel;
using System.Drawing;
namespace ControlsDemousing System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.ComponentModel;
using System.Drawing;
{
public class ExtdTextBox : TextBox
{
#region Member Variables
Color waterMarkColor = Color.Gray;
Color forecolor;
Font font;
Font waterMarkFont;
string waterMarkText = "Your Text Here";
#endregion
#region Constructor
public ExtdTextBox()
{
base.Text = this.waterMarkText;
this.forecolor = this.ForeColor;
this.ForeColor = this.waterMarkColor;
this.font = this.Font;
//event handlers
this.TextChanged += new EventHandler(ExtdTextBox_TextChanged);
this.KeyPress += new KeyPressEventHandler(ExtdTextBox_KeyPress);
this.LostFocus += new EventHandler(ExtdTextBox_TextChanged);
}
#endregion
#region Event Handler Methods
void ExtdTextBox_TextChanged(object sender, EventArgs e)
{
if (!string.IsNullOrEmpty(this.Text))
{
this.ForeColor = this.forecolor;
this.Font = this.font;
}
else
{
this.TextChanged -= new EventHandler(ExtdTextBox_TextChanged);
base.Text = this.waterMarkText;
this.TextChanged += new EventHandler(ExtdTextBox_TextChanged);
this.ForeColor = this.waterMarkColor;
this.Font = this.waterMarkFont;
}
}|
void ExtdTextBox_KeyPress(object sender, KeyPressEventArgs e)
{
string str = base.Text.Replace(this.waterMarkText, "");
this.TextChanged -= new EventHandler(ExtdTextBox_TextChanged);
this.Text = str;
this.TextChanged += new EventHandler(ExtdTextBox_TextChanged);
}
#endregion
#region User Defined Properties
/// <summary>
/// Property to set/get Watermark color at design/runtime
/// </summary>
[Browsable(true)]
[Category("Extended Properties")]
[Description("sets Watermark color")]
[DisplayName("WaterMark Color")]
public Color WaterMarkColor
{
get
{
return this.waterMarkColor;
}
set
{
this.waterMarkColor = value;
base.OnTextChanged(new EventArgs());
}
}
[Browsable(true)]
[Category("Extended Properties")]
[Description("sets TextBox text")]
[DisplayName("Text")]
/// <summary>
/// Property to get Text at runtime(hides base Text property)
/// </summary>
public new string Text
{
get
{
//required for validation for Text property
return base.Text.Replace(this.waterMarkText, string.Empty);
}
set
{
base.Text = value;
}
}
[Browsable(true)]
[Category("Extended Properties")]
[Description("sets WaterMark font")]
[DisplayName("WaterMark Font")]
/// <summary>
/// Property to get Text at runtime(hides base Text property)
/// </summary>
public Font WaterMarkFont
{
get
{
//required for validation for Text property
return this.waterMarkFont;
}
set
{
this.waterMarkFont = value;
this.OnTextChanged(new EventArgs());
}
}
/// <summary>
/// Property to set/get Watermark text at design/runtime
/// </summary>
[Browsable(true)]
[Category("Extended Properties")]
[Description("sets Watermark Text")]
[DisplayName("WaterMark Text")]
public string WaterMarkText
{
get
{
return this.waterMarkText;
}
set
{
this.waterMarkText = value;
base.OnTextChanged(new EventArgs());
}
}
#endregion
}
}
The other optional attributes declaration names are self-explanatory.
5) Build the project and refer to the dll in another Windows Forms project.
Right-click on the ToolBar Window and click ChooseItems:
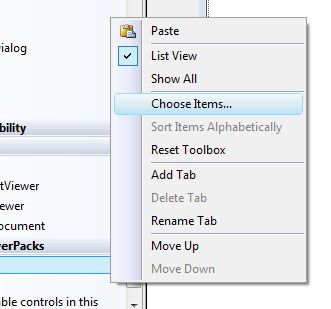
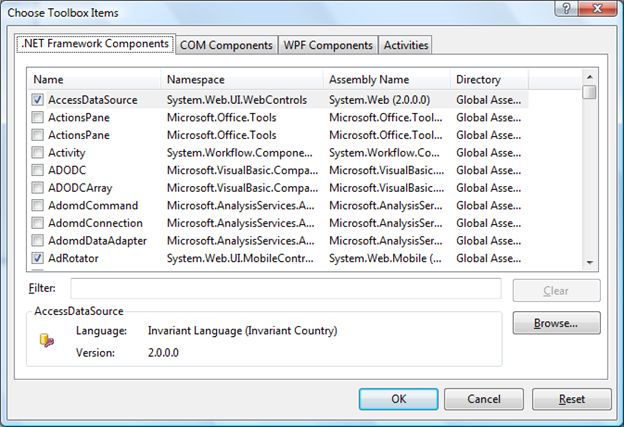
Click on browse and select control dll:
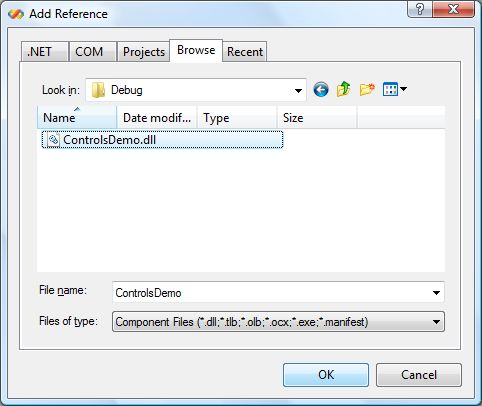
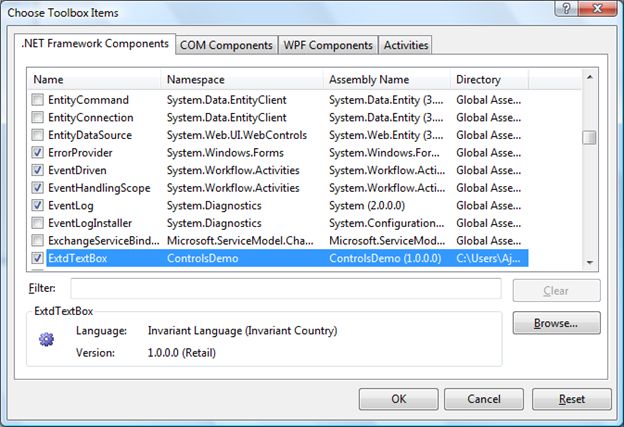
Click ok:
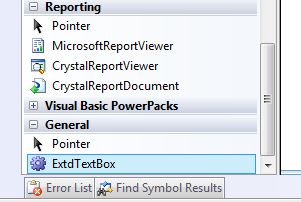
Drag and drop the control onto the form:
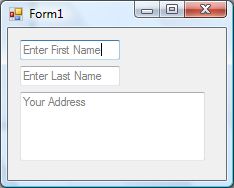
jquery modalpopup
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="jquerymodalpopup.aspx.cs" Inherits="Default21" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title></title>
<script type="text/javascript" src="http://code.jquery.com/jquery-1.6.4.min.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$('a.login-window').click(function () {
//Getting the variable's value from a link
var loginBox = $(this).attr('href');
//Fade in the Popup
$(loginBox).fadeIn(300);
//Set the center alignment padding + border see css style
var popMargTop = ($(loginBox).height() + 24) / 2;
var popMargLeft = ($(loginBox).width() + 24) / 2;
$(loginBox).css({
'margin-top': -popMargTop,
'margin-left': -popMargLeft
});
// Add the mask to body
$('body').append('<div id="mask"></div>');
$('#mask').fadeIn(300);
return false;
});
// When clicking on the button close or the mask layer the popup closed
$('a.close, #mask').live('click', function () {
$('#mask , .login-popup').fadeOut(300, function () {
$('#mask').remove();
});
return false;
});
});
</script>
<style type="text/css">
body
{
background:url(Images/bg.jpg) no-repeat #fff center top;
padding:0;
font-family:Arial, Helvetica, sans-serif;
font-size:11px;
margin:0px auto auto auto;
color:#000;
}
#mask {
display: none;
background: #000;
position: fixed; left: 0; top: 0;
z-index: 10;
width: 100%; height: 100%;
opacity: 0.6;
z-index: 999;
}
/* You can customize to your needs */
.login-popup{
display:none;
background-color:#FFFFFF;
padding: 10px;
border: 5px solid #BFBFBF;
float: left;
font-size: 1.2em;
position: fixed;
top: 50%; left: 50%;
z-index: 99999;
box-shadow: 0px 0px 20px #999; /* CSS3 */
-moz-box-shadow: 0px 0px 20px #999; /* Firefox */
-webkit-box-shadow: 0px 0px 20px #999; /* Safari, Chrome */
border-radius:3px 3px 3px 3px;
-moz-border-radius: 3px; /* Firefox */
-webkit-border-radius: 3px; /* Safari, Chrome */
}
</style>
</head>
<body>
<form id="form1" runat="server">
<div>
<a id="loginPopupFire" href="#login-box" rel="leanModal nofollow" class="login-window">Sign in</a>
<div id="login-box" class="login-popup">
<a href="#" class="close" style="float: right; text-decoration: none">X</a><br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa<br />
</div>
</div>
</form>
</body>
</html>
Saturday 27 October 2012
Thursday 25 October 2012
jquery form validation
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<link rel="stylesheet" href="style.css" type="text/css" media="screen" />
<script type="text/javascript"> var _siteRoot = 'index.html', _root = 'index.html';</script>
<script type="text/javascript" src="js/jquery.js"></script>
<script type="text/javascript" src="js/scripts.js"></script>
<script language="javascript" type="text/jscript">
$(document).ready
(
function () {
$("#<%=btn.ClientID %>").click
(
function () {
if ($("#<%=tb.ClientID %>").val() == "") {
alert('name should not blank.')
$("#<%=tb.ClientID %>").focus()
return false
}
else if ($("#<%=tb1.ClientID %>").val() == "") {
alert('Address should not blank.')
$("#<%=tb1.ClientID %>").focus()
return false
}
else if ($("#<%=rb.ClientID %>").is(":checked") == false && $("#<%=rb1.ClientID %>").is(":checked") == false) {
alert('Please select a gender.')
return false
}
else if ($("#<%=tb2.ClientID %>").val() == "") {
alert('Email id should not blank.')
$("#<%=tb2.ClientID %>").focus()
return false
}
else if ($("#<%=cb.ClientID %>").is(":checked") == false && $("#<%=cb1.ClientID %>").is(":checked") == false && $("#<%=cb2.ClientID %>").is(":checked") == false && $("#<%=cb3.ClientID %>").is(":checked") == false) {
alert('Please select a hobby.')
return false
}
else if ($("#<%=lb.ClientID %> :selected").length == 0) {
alert('Please select a interest.')
$("#<%=lb.ClientID %>").focus()
return false
}
else if ($("#<%=ddl.ClientID %>").get(0).selectedIndex == 0) {
alert('Please select a interest.')
$("#<%=ddl.ClientID %>").focus()
return false
}
else if ($("#<%=fu.ClientID %>").val()=="") {
alert('Please select a phote.')
return false
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=fu.ClientID %>").change
(
function () {
$("#<%=im.ClientID %>").attr("src", $("#<%=fu.ClientID %>").val())
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb.ClientID %>").keyup
(
function () {
var m = "~`!#$%^&*()-=+{}[]|:;\\\'\"<>,./?"
var n = $("#<%=tb.ClientID %>").val()
for (var i = 0; i < n.length; i++)
if (m.indexOf(n.charAt(i)) != -1) {
alert('You can not enter secial charecter.')
$("#<%=tb.ClientID %>").attr("value", "")
$("#<%=tb.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb2.ClientID %>").blur
(
function () {
var m = /\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*/
var n = $("#<%=tb2.ClientID %>").val()
if (n.search(m) == -1) {
alert('Invalid email id.')
$("#<%=tb2.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb1.ClientID %>").keyup
(
function () {
var m = event.keyCode
if(!(m<48 || m>57) || !(m<90 || m>105))
alert('This is a charecter field.')
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keyup
(
function () {
var m = event.keyCode
var n = "."
var p = $("#<%=tb5.ClientID %>").val()
for (var i = 0; i < p.length; i++)
if (n.indexOf(p.charAt(i)) == 0) {
var n = $("#<%=tb5.ClientID %>").val().split('.')
if (n[1].length > 2) {
alert('You can not enter more than 2 digits after decimal point')
$("#<%=tb5.ClientID %>").attr("value", n[0] + "." + n[1].substring(0, 2))
$("#<%=tb5.ClientID %>").focus()
}
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keydown
(
function () {
var m = event.keyCode
if (!(m < 65 || m > 90)) {
alert('This is a integer field.')
$("#<%=tb5.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb6.ClientID %>").keyup
(
function () {
var m = event.keyCode
if (!(m<65 || m>90)) {
alert('This is a integer field.')
$("#<%=tb6.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keypress
(
function () {
var m = event.keyCode
var n = $("#<%=tb5.ClientID %>").val()
if (m > 64 && m < 91) {
var q = ($("#<%=tb5.ClientID %>").val().length - 1)
var p = $("#<%=tb5.ClientID %>").val().substring(0, q)
$("#<%=tb5.ClientID %>").attr("value", p)
}
}
)
}
)
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<div id="header"><div class="wrap">
<div id="slide-holder">
<div id="slide-runner">
<a href=""><img id="slide-img-1" src="images/nature-photo.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-2" src="images/nature-photo1.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-3" src="images/nature-photo2.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-4" src="images/nature-photo3.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-5" src="images/nature-photo4.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-6" src="images/nature-photo4.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-7" src="images/nature-photo6.png" class="slide" alt="" /></a>
<div id="slide-controls">
<p id="slide-client" class="text"><strong>post: </strong><span></span></p>
<p id="slide-desc" class="text"></p>
<p id="slide-nav"></p>
</div>
</div>
<!--content featured gallery here -->
</div>
<script type="text/javascript">
if (!window.slider) var slider = {}; slider.data = [{ "id": "slide-img-1", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-2", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-3", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-4", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-5", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-6", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-7", "client": "siv sankar", "desc": "jay jagannath"}];
</script>
</div></div><!--/header-->
<center>
<div style="width:993px; height:500px; background-color:White; color:Black; font-family:Verdana">
<li class="x"> </li>
<li class="y"> </li>
<li class="z"><strong>EMPLOYEE INFORMATION</strong></li>
<li class="x">Name</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb" runat="server" Width="200"></asp:TextBox></li>
<li class="x" style="padding-top:10px">Address</li>
<li class="y" style="padding-top:10px">:</li>
<li class="z"><asp:TextBox ID="tb1" runat="server" Width="200" Height="30" TextMode="MultiLine"></asp:TextBox></li>
<li class="x">Gender</li>
<li class="y">:</li>
<li class="z"><asp:RadioButton ID="rb" runat="server" Text="Male" GroupName="a" /> <asp:RadioButton ID="rb1" runat="server" Text="Female" GroupName="a" /></li>
<li class="x">Email ID</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb2" runat="server" Width="200"></asp:TextBox></li>
<li class="x" style="padding-top:30px">Hobby</li>
<li class="y" style="padding-top:30px">:</li>
<li class="z"><fieldset style="width:198px"><asp:CheckBox ID="cb" runat="server" Text="Reading" /><br /><asp:CheckBox ID="cb1" runat="server" Text="Writing" /><br /><asp:CheckBox ID="cb2" runat="server" Text="Singing" /><br /><asp:CheckBox ID="cb3" runat="server" Text="Playing" /></fieldset></li>
<li class="x" style="padding-top:20px">Interest</li>
<li class="y" style="padding-top:20px">:</li>
<li class="z"><asp:ListBox ID="lb" runat="server" Rows="3" Width="200">
<asp:ListItem Text="Cricket"></asp:ListItem>
<asp:ListItem Text="Hockey"></asp:ListItem>
<asp:ListItem Text="Football"></asp:ListItem>
<asp:ListItem Text="Baseball"></asp:ListItem>
</asp:ListBox></li>
<li class="x">Salary</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb5" runat="server" Width="150"></asp:TextBox></li>
<li class="x">Question</li>
<li class="y">:</li>
<li class="z"><asp:DropDownList Width="200" ID="ddl" runat="server">
<asp:ListItem Text="--------Select--------"></asp:ListItem>
<asp:ListItem Text="What is ur name?"></asp:ListItem>
<asp:ListItem Text="What is ur favority place?"></asp:ListItem>
<asp:ListItem Text="What is ur favority song?"></asp:ListItem>
<asp:ListItem Text="What is ur favority movie?"></asp:ListItem>
</asp:DropDownList></li>
<li class="x">Age</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb6" runat="server" Width="100"></asp:TextBox></li>
<li class="x" style="padding-top:40px">Phote</li>
<li class="y" style="padding-top:40px">:</li>
<li class="z" style=" width:165px"><asp:Image ID="im" runat="server" Height="100" Width="100" /></li>
<li class="z" style=" width:365px; padding-top:40px "><asp:FileUpload ID="fu" runat="server" /></li>
<li class="x"> </li>
<li class="y"> </li>
<li class="z"><asp:Button ID="btn" runat="server" Text="Submit" Width="80" /> <asp:Button ID="Button1" runat="server" Text="Reset" Width="80" /></li>
</div>
</center>
</div>
</form>
</body>
</html>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<link rel="stylesheet" href="style.css" type="text/css" media="screen" />
<script type="text/javascript"> var _siteRoot = 'index.html', _root = 'index.html';</script>
<script type="text/javascript" src="js/jquery.js"></script>
<script type="text/javascript" src="js/scripts.js"></script>
<script language="javascript" type="text/jscript">
$(document).ready
(
function () {
$("#<%=btn.ClientID %>").click
(
function () {
if ($("#<%=tb.ClientID %>").val() == "") {
alert('name should not blank.')
$("#<%=tb.ClientID %>").focus()
return false
}
else if ($("#<%=tb1.ClientID %>").val() == "") {
alert('Address should not blank.')
$("#<%=tb1.ClientID %>").focus()
return false
}
else if ($("#<%=rb.ClientID %>").is(":checked") == false && $("#<%=rb1.ClientID %>").is(":checked") == false) {
alert('Please select a gender.')
return false
}
else if ($("#<%=tb2.ClientID %>").val() == "") {
alert('Email id should not blank.')
$("#<%=tb2.ClientID %>").focus()
return false
}
else if ($("#<%=cb.ClientID %>").is(":checked") == false && $("#<%=cb1.ClientID %>").is(":checked") == false && $("#<%=cb2.ClientID %>").is(":checked") == false && $("#<%=cb3.ClientID %>").is(":checked") == false) {
alert('Please select a hobby.')
return false
}
else if ($("#<%=lb.ClientID %> :selected").length == 0) {
alert('Please select a interest.')
$("#<%=lb.ClientID %>").focus()
return false
}
else if ($("#<%=ddl.ClientID %>").get(0).selectedIndex == 0) {
alert('Please select a interest.')
$("#<%=ddl.ClientID %>").focus()
return false
}
else if ($("#<%=fu.ClientID %>").val()=="") {
alert('Please select a phote.')
return false
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=fu.ClientID %>").change
(
function () {
$("#<%=im.ClientID %>").attr("src", $("#<%=fu.ClientID %>").val())
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb.ClientID %>").keyup
(
function () {
var m = "~`!#$%^&*()-=+{}[]|:;\\\'\"<>,./?"
var n = $("#<%=tb.ClientID %>").val()
for (var i = 0; i < n.length; i++)
if (m.indexOf(n.charAt(i)) != -1) {
alert('You can not enter secial charecter.')
$("#<%=tb.ClientID %>").attr("value", "")
$("#<%=tb.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb2.ClientID %>").blur
(
function () {
var m = /\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*/
var n = $("#<%=tb2.ClientID %>").val()
if (n.search(m) == -1) {
alert('Invalid email id.')
$("#<%=tb2.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb1.ClientID %>").keyup
(
function () {
var m = event.keyCode
if(!(m<48 || m>57) || !(m<90 || m>105))
alert('This is a charecter field.')
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keyup
(
function () {
var m = event.keyCode
var n = "."
var p = $("#<%=tb5.ClientID %>").val()
for (var i = 0; i < p.length; i++)
if (n.indexOf(p.charAt(i)) == 0) {
var n = $("#<%=tb5.ClientID %>").val().split('.')
if (n[1].length > 2) {
alert('You can not enter more than 2 digits after decimal point')
$("#<%=tb5.ClientID %>").attr("value", n[0] + "." + n[1].substring(0, 2))
$("#<%=tb5.ClientID %>").focus()
}
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keydown
(
function () {
var m = event.keyCode
if (!(m < 65 || m > 90)) {
alert('This is a integer field.')
$("#<%=tb5.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb6.ClientID %>").keyup
(
function () {
var m = event.keyCode
if (!(m<65 || m>90)) {
alert('This is a integer field.')
$("#<%=tb6.ClientID %>").focus()
}
}
)
}
)
$(document).ready
(
function () {
$("#<%=tb5.ClientID %>").keypress
(
function () {
var m = event.keyCode
var n = $("#<%=tb5.ClientID %>").val()
if (m > 64 && m < 91) {
var q = ($("#<%=tb5.ClientID %>").val().length - 1)
var p = $("#<%=tb5.ClientID %>").val().substring(0, q)
$("#<%=tb5.ClientID %>").attr("value", p)
}
}
)
}
)
</script>
</head>
<body>
<form id="form1" runat="server">
<div>
<div id="header"><div class="wrap">
<div id="slide-holder">
<div id="slide-runner">
<a href=""><img id="slide-img-1" src="images/nature-photo.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-2" src="images/nature-photo1.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-3" src="images/nature-photo2.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-4" src="images/nature-photo3.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-5" src="images/nature-photo4.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-6" src="images/nature-photo4.png" class="slide" alt="" /></a>
<a href=""><img id="slide-img-7" src="images/nature-photo6.png" class="slide" alt="" /></a>
<div id="slide-controls">
<p id="slide-client" class="text"><strong>post: </strong><span></span></p>
<p id="slide-desc" class="text"></p>
<p id="slide-nav"></p>
</div>
</div>
<!--content featured gallery here -->
</div>
<script type="text/javascript">
if (!window.slider) var slider = {}; slider.data = [{ "id": "slide-img-1", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-2", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-3", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-4", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-5", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-6", "client": "siv sankar", "desc": "jay jagannath" }, { "id": "slide-img-7", "client": "siv sankar", "desc": "jay jagannath"}];
</script>
</div></div><!--/header-->
<center>
<div style="width:993px; height:500px; background-color:White; color:Black; font-family:Verdana">
<li class="x"> </li>
<li class="y"> </li>
<li class="z"><strong>EMPLOYEE INFORMATION</strong></li>
<li class="x">Name</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb" runat="server" Width="200"></asp:TextBox></li>
<li class="x" style="padding-top:10px">Address</li>
<li class="y" style="padding-top:10px">:</li>
<li class="z"><asp:TextBox ID="tb1" runat="server" Width="200" Height="30" TextMode="MultiLine"></asp:TextBox></li>
<li class="x">Gender</li>
<li class="y">:</li>
<li class="z"><asp:RadioButton ID="rb" runat="server" Text="Male" GroupName="a" /> <asp:RadioButton ID="rb1" runat="server" Text="Female" GroupName="a" /></li>
<li class="x">Email ID</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb2" runat="server" Width="200"></asp:TextBox></li>
<li class="x" style="padding-top:30px">Hobby</li>
<li class="y" style="padding-top:30px">:</li>
<li class="z"><fieldset style="width:198px"><asp:CheckBox ID="cb" runat="server" Text="Reading" /><br /><asp:CheckBox ID="cb1" runat="server" Text="Writing" /><br /><asp:CheckBox ID="cb2" runat="server" Text="Singing" /><br /><asp:CheckBox ID="cb3" runat="server" Text="Playing" /></fieldset></li>
<li class="x" style="padding-top:20px">Interest</li>
<li class="y" style="padding-top:20px">:</li>
<li class="z"><asp:ListBox ID="lb" runat="server" Rows="3" Width="200">
<asp:ListItem Text="Cricket"></asp:ListItem>
<asp:ListItem Text="Hockey"></asp:ListItem>
<asp:ListItem Text="Football"></asp:ListItem>
<asp:ListItem Text="Baseball"></asp:ListItem>
</asp:ListBox></li>
<li class="x">Salary</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb5" runat="server" Width="150"></asp:TextBox></li>
<li class="x">Question</li>
<li class="y">:</li>
<li class="z"><asp:DropDownList Width="200" ID="ddl" runat="server">
<asp:ListItem Text="--------Select--------"></asp:ListItem>
<asp:ListItem Text="What is ur name?"></asp:ListItem>
<asp:ListItem Text="What is ur favority place?"></asp:ListItem>
<asp:ListItem Text="What is ur favority song?"></asp:ListItem>
<asp:ListItem Text="What is ur favority movie?"></asp:ListItem>
</asp:DropDownList></li>
<li class="x">Age</li>
<li class="y">:</li>
<li class="z"><asp:TextBox ID="tb6" runat="server" Width="100"></asp:TextBox></li>
<li class="x" style="padding-top:40px">Phote</li>
<li class="y" style="padding-top:40px">:</li>
<li class="z" style=" width:165px"><asp:Image ID="im" runat="server" Height="100" Width="100" /></li>
<li class="z" style=" width:365px; padding-top:40px "><asp:FileUpload ID="fu" runat="server" /></li>
<li class="x"> </li>
<li class="y"> </li>
<li class="z"><asp:Button ID="btn" runat="server" Text="Submit" Width="80" /> <asp:Button ID="Button1" runat="server" Text="Reset" Width="80" /></li>
</div>
</center>
</div>
</form>
</body>
</html>
SQL Server - Differences between Functions and Stored Procedures
Stored Procedre:
3) Procedure allows select as well as DML(INSERT/UPDATE/DELETE) statements in it whereas function allows only select statement in it.
4) Functions can be called from procedure whereas procedures cannot be called from function.
5) Exception can be handled by try-catch block in a procedure whereas try-catch block cannot be used in a function.
6) We can go for transaction management in procedure whereas we can't go in function.
7) Procedures cannot be utilized in a select statement whereas function can be embedded in a select statement.
Stored
Procedure is a group of sql statements that has been created once and stored in
server database. It’s pre-compile
objects which are compiled for first time and its compiled format is saved
which executes (compiled code) whenever it is called. Stored procedures
will accept input parameters so that single stored procedure can be used over
network by multiple clients using different input data. Stored procedures will
reduce network traffic and increase the performance.
Function:
Function is not pre-compiled object it will execute every
time whenever it was called.
Difference between Stored Procedure and
Function
2)
Procedures can have input, output parameters for it whereas functions can have
only input parameters.
3) Procedure allows select as well as DML(INSERT/UPDATE/DELETE) statements in it whereas function allows only select statement in it.
4) Functions can be called from procedure whereas procedures cannot be called from function.
5) Exception can be handled by try-catch block in a procedure whereas try-catch block cannot be used in a function.
6) We can go for transaction management in procedure whereas we can't go in function.
7) Procedures cannot be utilized in a select statement whereas function can be embedded in a select statement.
how to return multiple values from stored procedure in sql server
ALTER PROCEDURE p3
(
@EID INT,
@NAME VARCHAR(50) OUTPUT,
@SAL MONEY OUTPUT
)
AS
begin
select name,sal from emp where eid=@EID
end
EXECUTE
DECLARE @NAME VARCHAR(50),@SAL MONEY
EXEC P3 1,@NAME=@NAME OUTPUT,@SAL=@SAL
(
@EID INT,
@NAME VARCHAR(50) OUTPUT,
@SAL MONEY OUTPUT
)
AS
begin
select name,sal from emp where eid=@EID
end
EXECUTE
DECLARE @NAME VARCHAR(50),@SAL MONEY
EXEC P3 1,@NAME=@NAME OUTPUT,@SAL=@SAL
Friday 19 October 2012
how to save image in folder in windows c#.net
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication11
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
string s = string.Empty;
private void Form2_Load(object sender, EventArgs e)
{
s = "Chrysanthemum.jpg";
}
private void textBox2_TextChanged(object sender, EventArgs e)
{
}
private void save_Click(object sender, EventArgs e)
{
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "Resources\\" + textBox2.Text;
Image img = Image.FromFile(s);
img.Save(GetImage, System.Drawing.Imaging.ImageFormat.Jpeg);
// txtFilename.Text = openDiag.FileName.ToString();
// pictureBox1.Image = Image.FromFile(txtFilename.Text.Trim());
// string x = pictureBox1.ImageLocation;
//string ImagePath = Application.StartupPath;
//pnlCommon.BackgroundImage = Image.FromFile("" + ImagePath + "Resources/" + imagenamePL);
//Image img = Image.FromFile(TxtDesignImage.Text);
//img.Save(Application.StartupPath + "\\ItemImage\\" + CurrentFileName, System.Drawing.Imaging.ImageFormat.Jpeg);
}
private void button1_Click(object sender, EventArgs e)
{
try
{
OpenFileDialog open = new OpenFileDialog();
open.Filter = "Image Files(*.jpg; *.jpeg; *.gif; *.bmp)|*.jpg; *.jpeg; *.gif; *.bmp";
if (open.ShowDialog() == DialogResult.OK)
{
pictureBox1.Image = Image.FromFile(open.FileName);
s = open.FileName;
textBox2.Text = open.SafeFileName.ToString();
}
}
catch (Exception)
{
throw new ApplicationException("Image loading error....");
}
}
private void RemoveImage()
{
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "ItemImage\\" + s;
System.IO.File.Delete(GetImage);
}
private void Display_Click(object sender, EventArgs e)
{
//Resources FOLDER
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "\\Resources\\" + s;
pictureBox1.Image = Image.FromFile(GetImage);
//OTHER FOLDER
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
//string[] words = GetImage.Split('.');
//GetImage = words[0] + GetUniqueKey() + "." + words[1];
string serverpath = "FileUploads\\CardType\\" + FileName;
GetImage += serverpath;
Image img = Image.FromFile(FileFullPath);
img.Save(GetImage, System.Drawing.Imaging.ImageFormat.Jpeg);
newCardTYpe.Image = serverpath;
ERPManagement.GetInstance.InsertCardType(newCardTYpe);
MessageBox.Show("Data saved successfully.", "KenCloud", MessageBoxButtons.OK, MessageBoxIcon.Information);
TxtCardTypeName.Focus();
}
private void button4_Click(object sender, EventArgs e)
{
pictureBox1.Image = Bitmap.FromFile(s);
}
}
}
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
namespace WindowsFormsApplication11
{
public partial class Form2 : Form
{
public Form2()
{
InitializeComponent();
}
string s = string.Empty;
private void Form2_Load(object sender, EventArgs e)
{
s = "Chrysanthemum.jpg";
}
private void textBox2_TextChanged(object sender, EventArgs e)
{
}
private void save_Click(object sender, EventArgs e)
{
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "Resources\\" + textBox2.Text;
Image img = Image.FromFile(s);
img.Save(GetImage, System.Drawing.Imaging.ImageFormat.Jpeg);
// txtFilename.Text = openDiag.FileName.ToString();
// pictureBox1.Image = Image.FromFile(txtFilename.Text.Trim());
// string x = pictureBox1.ImageLocation;
//string ImagePath = Application.StartupPath;
//pnlCommon.BackgroundImage = Image.FromFile("" + ImagePath + "Resources/" + imagenamePL);
//Image img = Image.FromFile(TxtDesignImage.Text);
//img.Save(Application.StartupPath + "\\ItemImage\\" + CurrentFileName, System.Drawing.Imaging.ImageFormat.Jpeg);
}
private void button1_Click(object sender, EventArgs e)
{
try
{
OpenFileDialog open = new OpenFileDialog();
open.Filter = "Image Files(*.jpg; *.jpeg; *.gif; *.bmp)|*.jpg; *.jpeg; *.gif; *.bmp";
if (open.ShowDialog() == DialogResult.OK)
{
pictureBox1.Image = Image.FromFile(open.FileName);
s = open.FileName;
textBox2.Text = open.SafeFileName.ToString();
}
}
catch (Exception)
{
throw new ApplicationException("Image loading error....");
}
}
private void RemoveImage()
{
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "ItemImage\\" + s;
System.IO.File.Delete(GetImage);
}
private void Display_Click(object sender, EventArgs e)
{
//Resources FOLDER
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
GetImage += "\\Resources\\" + s;
pictureBox1.Image = Image.FromFile(GetImage);
//OTHER FOLDER
string GetImage = (System.Environment.CurrentDirectory);
GetImage = GetImage.Substring(0, GetImage.Length - GetImage.StartsWith("bin").ToString().Length - 4);
//string[] words = GetImage.Split('.');
//GetImage = words[0] + GetUniqueKey() + "." + words[1];
string serverpath = "FileUploads\\CardType\\" + FileName;
GetImage += serverpath;
Image img = Image.FromFile(FileFullPath);
img.Save(GetImage, System.Drawing.Imaging.ImageFormat.Jpeg);
newCardTYpe.Image = serverpath;
ERPManagement.GetInstance.InsertCardType(newCardTYpe);
MessageBox.Show("Data saved successfully.", "KenCloud", MessageBoxButtons.OK, MessageBoxIcon.Information);
TxtCardTypeName.Focus();
}
private void button4_Click(object sender, EventArgs e)
{
pictureBox1.Image = Bitmap.FromFile(s);
}
}
}
Thursday 18 October 2012
how to save image in data base in windows c#.net
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
using System.Data.SqlClient;
namespace FileHandlingExp
{
public partial class StoreImageInDataBase : Form
{
public
StoreImageInDataBase()
{
InitializeComponent();
}
private
void StoreImageInDataBase_Load(object sender, EventArgs
e)
{
}
object
objimage;
SqlDataReader
dr;
//Code for select image
from any Drive……………………………………………
private
void btnSelectImage_Click(object sender, EventArgs
e)
{
if
(openFileDialog1.ShowDialog() == DialogResult.OK)
{
txtimagesource.Text =
openFileDialog1.FileName;
}
}
//Code for save image in table in binary format
private
void btnSaveImage_Click(object sender, EventArgs e)
{
String sBLOBFilePath =s;
FileStream fsBLOBFile = new FileStream(sBLOBFilePath, FileMode.Open, FileAccess.Read);
Byte[] bytBLOBData = new Byte[fsBLOBFile.Length];
fsBLOBFile.Read(bytBLOBData, 0, bytBLOBData.Length);
fsBLOBFile.Close();
obj.Item_Img = bytBLOBData;
FileStream fsBLOBFile = new FileStream(sBLOBFilePath, FileMode.Open, FileAccess.Read);
Byte[] bytBLOBData = new Byte[fsBLOBFile.Length];
fsBLOBFile.Read(bytBLOBData, 0, bytBLOBData.Length);
fsBLOBFile.Close();
obj.Item_Img = bytBLOBData;
SqlConnection
con = new SqlConnection("Data Source=KUSH-PC;Initial Catalog=test;Integrated
Security=True");
SqlCommand
com = new SqlCommand("insert into TableImage(Id,Name,UserImage)
values(@id,@name,@image)", con);
com.Parameters.AddWithValue("@id",Convert.ToInt32(txtid.Text));
com.Parameters.AddWithValue("@name", txtname.Text);
com.Parameters.AddWithValue("@image", bytBLOBData);
con.Open();
com.ExecuteNonQuery();
con.Close();
MessageBox.Show("Image Added");
}
//Code for show image(binary format) in PictureBox from table …………………………
private
void btnLoadImage_Click(object sender, EventArgs e)
{
SqlConnection
con = new SqlConnection("Data Source=KUSH-PC;Initial Catalog=test;Integrated
Security=True");
SqlCommand
com = new SqlCommand("select Id,Name,UserImage from TableImage where
Id=@id", con);
com.Parameters.AddWithValue("@id", Convert.ToInt32(txtid.Text));
con.Open();
dr = com.ExecuteReader();
if
(dr.HasRows)
{
dr.Read();
txtname.Text= ""+dr["Name"];
objimage = dr["UserImage"];
}
//objimage
= com.ExecuteScalar();
byte[]
data;
data = (byte[])objimage;
MemoryStream
ms = new MemoryStream(data);
pictureBox1.Image = Image.FromStream(ms);
con.Close();
}
//Code for go to next
page…………………………………………
private
void btnNextPage_Click(object
sender, EventArgs e)
{
CopyFile
cf = new CopyFile();
cf.Show();
}
}
}
After retrieve image
from table image is stored
in binary format and after retrieve
image show in PictureBox Control in Windows Application
in C# .NET
How to copy
image from another
location from computer drive and
paste in other drive in computer in Windows Application in C# .NET
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Windows.Forms;
using System.IO;
namespace FileHandlingExp
{
public partial class CopyFile : Form
{
public
CopyFile()
{
InitializeComponent();
}
// Code for
next page
private
void btnNext_Click(object
sender, EventArgs e)
{
StoreImageInDataBase
si = new StoreImageInDataBase();
si.Show();
}
// Code for how to
select image from Computer drive …………..
private
void btnSelectSource_Click(object sender, EventArgs
e)
{
if
(openFileDialog1.ShowDialog() == DialogResult.OK)
{
txtsourceaddress.Text =
openFileDialog1.FileName;
}
}
// Code for copy image
where is paste in Computer drive …………..
private
void btnLocateSource_Click(object sender, EventArgs
e)
{
if
(saveFileDialog1.ShowDialog() == DialogResult.OK)
{
txtdestinationaddress.Text =
saveFileDialog1.FileName;
}
}
// Code for how to
save image ……………………………………
private
void btnCopyPaste_Click(object sender, EventArgs e)
{
FileStream
fssource = new FileStream(txtsourceaddress.Text,
FileMode.Open);
FileStream
fsdest = new FileStream(txtdestinationaddress.Text,
FileMode.Create);
while
(true)
{
int
i;
i = fssource.ReadByte();
if
(i == -1)
break;
fsdest.WriteByte(Convert.ToByte(i));
}
fsdest.Close();
MessageBox.Show("Image copy in Location");
}
}
}
Subscribe to:
Posts (Atom)